Here is a short code example that builds on the previous post “How to show a popup once per day.” In this case, we want to show the popup after the user scrolled down 950 pixels (instead of a 3-second delay)
Table of Contents
The Full Snippet
<script>
(function(){
var distance = 950; // Scroll distance in pixel.
var expireKey = 'newsletter'; // Name of the localStorage timestamp.
var popupId = '#get-newsletter'; // ID of the popup to display.
function maybeShowPopup() {
// Check if the user scrolled far enough.
if ( jQuery(window).scrollTop() < distance ) {
return;
}
// Remove the custom scroll event, to avoid displaying the popup multiple times.
jQuery( window ).off( '.popup_' + expireKey );
// Check, if the popup was displayed already today.
if ( window.localStorage ) {
var nextPopup = localStorage.getItem( expireKey );
if (nextPopup > new Date()) {
return;
}
var expires = new Date();
expires = expires.setHours(expires.getHours() + 24);
localStorage.setItem( expireKey, expires );
}
DiviPopup.openPopup( popupId );
}
// Attach the scroll listener to the window.
jQuery(window).on( 'scroll.popup_' + expireKey, maybeShowPopup );
})()
</script>
Some Notes
As you see, we pick the localStorage solution and not the Cookie option to limit the popup to once per day. This is a personal preference.
In this example, we have three variables at the top. This makes it easy for us (or you) to copy the snippet to various projects and only adjust those three variables to get your desired popup logic.
Also, you can see that we attach the event listener using 'scroll.popup_' + expireKey
. The part after the dot (.) is the namespace of the event: 'popoup_newsletter'
, which allows us to completely remove the event. It’s not strictly necessary in this scenario, but a good example ๐
*Related Article – Divi vs Elementor: Which Page Builder Is the Best in 2024
Another thing you can see in the snippet is the surrounding code: (function(){ ... })()
– this is called IIFE, or Immediately Invoked Function Expression. This expression makes sure, that your code is “private” and does not drip into the global scope where it could cause conflicts with other libraries or plugins.
Btw: It’s safe to use the same snippet multiple times on the same page. Just remember to modify the variables at the top, and everything will be fine!
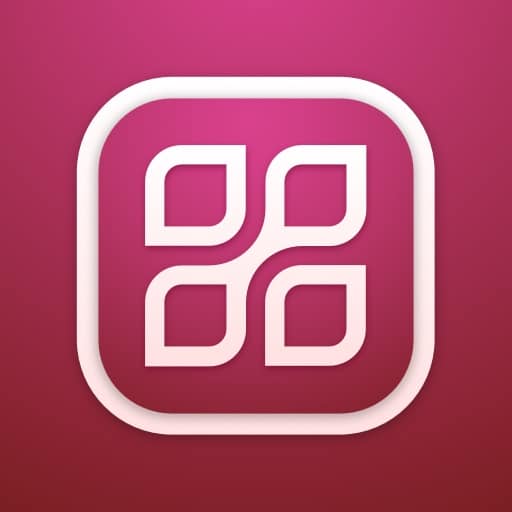
Try Divi Areas Pro today
Sounds interesting? Learn more about Divi Areas Pro and download your copy now!
Many pre-designed layouts. Automated triggers. No coding.
Click here for more details